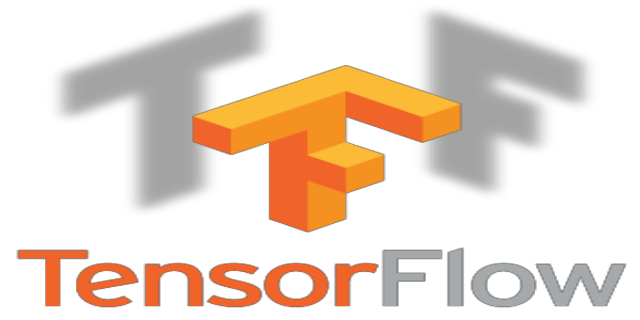
TensorFlow is an open-source machine learning software built by Google to train neural networks. TensorFlow's neural networks are expressed in the form of stateful dataflow graphs. Each node in the graph represents the operations performed by neural networks on multi-dimensional arrays. These multi-dimensional arrays are commonly known as "tensors", hence the name TensorFlow.
TensorFlow is a deep learning software system. It works well for information retrieval, as demonstrated by Google in how they do search ranking in their machine-learning artificial intelligence system, RankBrain. TensorFlow can perform image recognition, as shown in Google's Inception, as well as human language audio recognition. It's also useful in solving other problems not specific to machine learning, such as partial differential equations.
The TensorFlow architecture allows for deployment on multiple CPUs or GPUs within a desktop, server or mobile device. There are also extensions for integration with CUDA, a parallel computing platform from Nvidia. This gives users who are deploying on a GPU direct access to the virtual instruction set and other elements of the GPU that are necessary for parallel computational tasks.
In this guide, we'll install TensorFlow's "CPU support only" version. This set up is ideal for people looking to install and use TensorFlow, but who don't have an Nvidia graphics card or don't need to run performance-critical applications.
These steps can also be performed on earlier release of Ubuntu.
You can install TensorFlow several ways. Each method has a different use case and development environment:
- Python and Virtualenv: In this procedure, you install TensorFlow and all of the packages required to use TensorFlow in a Python virtual environment. This isolates your TensorFlow environemnt from other Python programs on the same machine.
- Native pip: In this method, you install TensorFlow on your system globally. This is recommended for people who want to make TensorFlow available to everyone on a multi-user system. This method of installation does not isolate TensorFlow in a contained environment and may interfere with other Python installations or libraries.
- Docker: Docker is a container runtime environment and completely isolates its contents from preexisting packages on your system. In this method, you use a Docker container that contains TensorFlow and all of its dependencies. This method is ideal for incorporating TensorFlow into a larger application architecture already using Docker. However, the size of the Docker image will be quite large.
We'll install TensorFlow in a Python virtual environment with virtualenv. This method isolates the TensorFlow installation and gets things up and running quickly. Once you complete the installation, you'll validate your installation by running a short TensorFlow program and then use TensorFlow to perform image recognition.
Prerequisites
Before you begin this guide, you'll need the following:- One Ubuntu 17.10 server with at least 1GB of RAM set up by following the Ubuntu 17.10 initial server setup guide, including a sudo non-root user and a firewall.
- Python 3.x or higher and virtualenv installed.
- Git installed.
Setting Up Python 3
Ubuntu 17.10 comes with both Python 3 and Python 2 pre-installed. To make sure that your releases are up-to-date, let’s update and upgrade the system with apt-get:
sudo apt-get update
sudo apt-get -y upgrade
Once the process is complete, we can check the version of Python 3 that is installed in the system by typing:
python3 -V
Output
Python 3.6.3
To manage software packages for Python, let’s install pip:
sudo apt-get install -y python3-pip
A tool for use with Python, pip installs and manages programming packages we may want to use in our development projects. You can install Python packages by typing:
pip3 install package_name
Here, package_name can refer to any Python package or library, such as Django for web development or NumPy for scientific computing. So if you would like to install NumPy, you can do so with the command pip3 install numpy.
There are a few more packages and development tools to install to ensure that we have a robust set-up for our programming environment:
sudo apt-get install build-essential libssl-dev libffi-dev python-dev
Once Python is set up, and pip and other tools are installed, we can set up a virtual environment for our development projects.
Setting Up a Virtual Environment
Virtual environments enable you to have an isolated space on your computer for Python projects, ensuring that each of your projects can have its own set of dependencies that won’t disrupt any of your other projects.You can set up as many Python programming environments as you want. Each environment is basically a directory or folder in your computer that has a few scripts in it to make it act as an environment.
We need to first install the venv module, part of the standard Python 3 library, so that we can create virtual environments. Let’s install venv by typing:
sudo apt-get install -y python3-venv
With this installed, we are ready to create environments. Let’s choose which directory we would like to put our Python programming environments in, or we can create a new directory with mkdir, as in:
mkdir environments
cd environments
Once you are in the directory where you would like the environments to live, you can create an environment by running the following command:
python3 -m venv my_env
ls my_env
Output
bin include lib lib64 pyvenv.cfg share
To use this environment, you need to activate it, which you can do by typing the following command that calls the activate script:
source my_env/bin/activate
Your prompt will now be prefixed with the name of your environment, in this case it is called my_env. Your prefix may look somewhat different, but the name of your environment in parentheses should be the first thing you see on your line:
(my_env) username@hostname:~/environments$
This prefix lets us know that the environment my_env is currently active, meaning that when we create programs here they will use only this particular environment’s settings and packages.
Note: Within the virtual environment, you can use the command python instead of python3, and pip instead of pip3 if you would prefer. If you use Python 3 on your machine outside of an environment, you will need to use the python3 and pip3 commands exclusively.
After following these steps, your virtual environment is ready to use.
Creating a Simple Program
Now that we have our virtual environment set up, let’s create a simple “Hello, World!” program. This will make sure that our environment is working and gives us the opportunity to become more familiar with Python if we aren’t already.
To do this, we’ll open up a command-line text editor such as nano and create a new file:
nano hello.py
Once the text file opens up in the terminal window we’ll type out our program:
print("Hello, World!")
Exit nano by typing the control and x keys, and when prompted to save the file press y.
Once you exit out of nano and return to your shell, let’s run the program:
python hello.py
The hello.py program that you just created should cause your terminal to produce the following output:
Output
Hello, World!
To leave the environment, simply type the command deactivate and you will return to your original directory.
Congratulations! At this point you have a Python 3 programming environment set up on your local Ubuntu machine and can begin with the following!
Installing Git
You can use the apt package management tools to update your local package index. Afterwards, you can download and install the program:
sudo apt-get update
sudo apt-get install git
This will download and install git to your system.
Set Up Git
Now that you have git installed, you need to do a few things so that the commit messages that will be generated for you will contain your correct information.
The easiest way of doing this is through the git config command. Specifically, we need to provide our name and email address because git embeds this information into each commit we do. We can go ahead and add this information by typing:
git config --global user.name "Your Name"
git config --global user.email "youremail@domain.com"
We can see all of the configuration items that have been set by typing:
git config --list
user.name=Your Name
user.email=youremail@domain.com
As you can see, this has a slightly different format. The information is stored in your git configuration file, which you can optionally edit by hand with your text editor like this:
nano ~/.gitconfig
[user]
name = Your Name
email = youremail@domain.com
There are many other options that you can set, but these are the two essential ones needed. If you skip this step, you'll likely see warnings when you commit to git that are similar to this:
Output when git username and email not set
[master 0d9d21d] initial project version
Committer: root
Your name and email address were configured automatically based
on your username and hostname. Please check that they are accurate.
You can suppress this message by setting them explicitly:
git config --global user.name "Your Name"
git config --global user.email you@example.com
After doing this, you may fix the identity used for this commit with:
git commit --amend --reset-author
This makes more work for you because you will then have to revise the commits you have done with the corrected information.
You should now have git installed and ready to use on your Ubuntu system.
Installing TensorFlow
In this step we are going to create a virtual environment and install TensorFlow.First, create a project directory called tf-setup:
mkdir ~/tf-setup
Navigate to your newly created tf-demo directory:
cd ~/tf-setup
Then create a new virtual environment called tensorflow-dev. Run the following command to create the environment:
python3 -m venv tensorflow-dev
This creates a new tensorflow-dev directory which will contain all of the packages that you install while this environment is activated. It also includes pip and a standalone version of Python.
Now activate your virtual environment:
source tensorflow-dev/bin/activate
Once activated, you will see something similar to this in your terminal:
(tensorflow-dev)username@hostname:~/tf-setup $
Now you can install TensorFlow in your virtual environment.
Run the following command to install and upgrade to the newest version of TensorFlow available in PyPi:
pip3 install --upgrade tensorflow
TensorFlow will install:
Output
Collecting tensorflow
Downloading tensorflow-1.4.0-cp36-cp36m-macosx_10_11_x86_64.whl (39.3MB)
100% |████████████████████████████████| 39.3MB 35kB/s
...
Successfully installed bleach-1.5.0 enum34-1.1.6 html5lib-0.9999999 markdown-2.6.9 numpy-1.13.3 protobuf-3.5.0.post1 setuptools-38.2.3 six-1.11.0 tensorflow-1.4.0 tensorflow-tensorboard-0.4.0rc3 werkzeug-0.12.2 wheel-0.30.0
If you'd like to deactivate your virtual environment at any time, the command is:
deactivate
To reactivate the environment later, navigate to your project directory and run source tensorflow-dev/bin/activate.
Now, that you have installed TensorFlow, let's make sure the TensorFlow installation works.
Validating Installation
To validate the installation of TensorFlow, we are going to run a simple program in TensorFlow as a non-root user. We will use the canonical beginner's example of "Hello, world!" as a form of validation. Rather than creating a Python file, we'll create this program using Python's interactive console.To write the program, start up your Python interpreter:
python
You will see the following prompt appear in your terminal
>>>
This is the prompt for the Python interpreter, and it indicates that it's ready for you to start entering some Python statements.
First, type this line to import the TensorFlow package and make it available as the local variable tf. Press ENTER after typing in the line of code:
import tensorflow as tf
Next, add this line of code to set the message "Hello, world!":
hello = tf.constant("Hello, world!")
Then create a new TensorFlow session and assign it to the variable sess:
sess = tf.Session()
Note: Depending on your environment, you might see this output:
Output
2017-11-04 16:22:45.956946: M tensorflow/core/platform/cpu_feature_guard.cc:45] The TensorFlow library wasn't compiled to use SSE4.1 instructions, but these are available on your machine and could speed up CPU computations.
2017-11-04 16:22:45.957158: M tensorflow/core/platform/cpu_feature_guard.cc:45] The TensorFlow library wasn't compiled to use SSE4.2 instructions, but these are available on your machine and could speed up CPU computations.
2017-11-04 16:22:45.957282: M tensorflow/core/platform/cpu_feature_guard.cc:45] The TensorFlow library wasn't compiled to use AVX instructions, but these are available on your machine and could speed up CPU computations.
2017-11-04 16:22:45.957404: M tensorflow/core/platform/cpu_feature_guard.cc:45] The TensorFlow library wasn't compiled to use AVX2 instructions, but these are available on your machine and could speed up CPU computations.
2017-11-04 09:30:50.757527: M tensorflow/core/platform/cpu_feature_guard.cc:45]
The TensorFlow library wasn't compiled to use FMA instructions, but these are available on your machine and could speed up CPU computations.
This tells you that that you have an instruction set that has the potential to be optimized for better performance with TensorFlow. If you see this, you can safely ignore it and continue.
Finally, enter this line of code to print out the result of running the hello TensorFlow session you've constructed in your previous lines of code:
print(sess.run(hello))
You'll see this output in your console:
Output
Hello, world!
This indicates that everything is working and that you can start using TensorFlow to do something more interesting.
Exit the Python interactive console by pressing CTRL+D.
Now let's use TensorFlow's image recognition API to get more familiar with TensorFlow.
Using TensorFlow for Image Recognition
Now that TensorFlow is installed and you've validated it by running a simple program, let's look at TensorFlow's image recognition capabilities.In order to classify an image you need to train a model. Then you need to write some code to use the model.
TensorFlow provides a repository of models and examples, including code and a trained model for classifying images.
Use Git to clone the TensorFlow models repository from GitHub into your project directory:
git clone https://github.com/tensorflow/models.git
You will see the following output as Git checks out the repository into a new folder called models:
Output
Cloning into 'models'...
remote: Counting objects: 8785, done.
remote: Total 8785 (delta 0), reused 0 (delta 0), pack-reused 8785
Receiving objects: 100% (8785/8785), 203.16 MiB | 24.16 MiB/s, done.
Resolving deltas: 100% (4942/4942), done.
Checking connectivity... done.
Switch to the models/tutorials/image/imagenet directory:
cd models/tutorials/image/imagenet
This directory contains the classify_image.py file which uses TensorFlow to recognize images. This program downloads a trained model from tensorflow.org on its first run. Downloading this model requires that you have 200MB of free space available on disk.
In this example, we will classify a pre-supplied image of a Panda. Execute this command to run the image classifier program:
python classify_image.py
You'll see output similar to this:
Output
giant panda, panda, panda bear, coon bear, Ailuropoda melanoleuca (score = 0.89107)
indri, indris, Indri indri, Indri brevicaudatus (score = 0.00779)
lesser panda, red panda, panda, bear cat, cat bear, Ailurus fulgens (score = 0.00296)
custard apple (score = 0.00147)
earthstar (score = 0.00117)
You have classified your first image using the image recognition capabilities of TensorFlow.
If you'd like to use another image, you can do this by adding the -- image_file argument to your python3 classify_image.py command. For the argument, you'd pass in the absolute path of the image file.