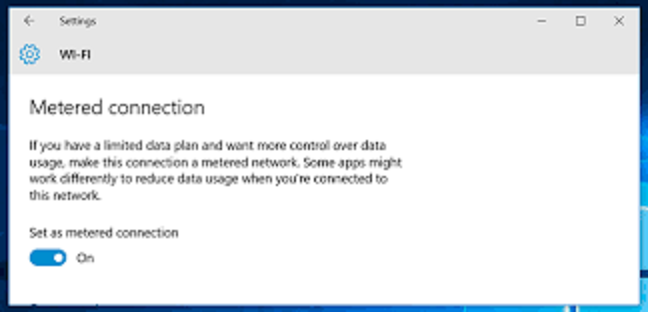
If you set your internet connection to metered, Windows will limit automatic downloads such as Windows Update. Whereas a Wi-Fi connection can be set to a metered connection easily with a few mouse clicks, things are a bit more complicated with an Ethernet connection. I wrote a little PowerShell script that allows quick switching between metered and not metered connections.
Unfortunately, the procedure to set an Ethernet connection as metered is quite long-winded, because, by default, Administrators don’t have the right to change the corresponding Registry key. For the sake of completeness, I show you how to do it with the Registry editor. But if you want to avoid all this click-click, you can simply run the PowerShell script mentioned in the end of this post.
- Run Registry editor (Windows key + R, type regedit, click OK)
- Navigate to HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\NetworkList\DefaultMediaCost
- Right click DefaultMediaCost, select Permissions, and click Advanced.
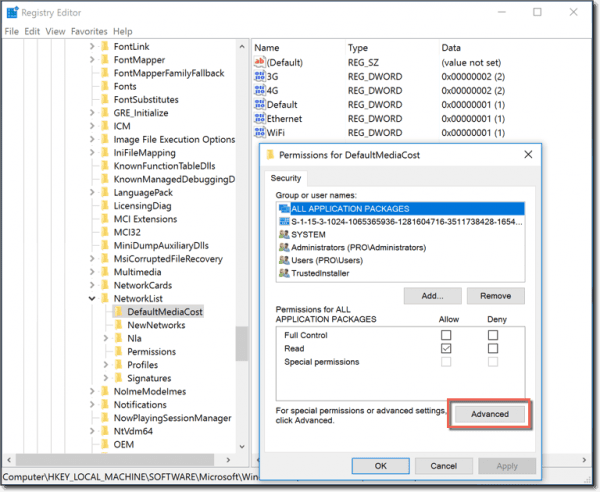
Change Permissions on DefaultMediaCost key
Click Change to assign a different owner for the key.
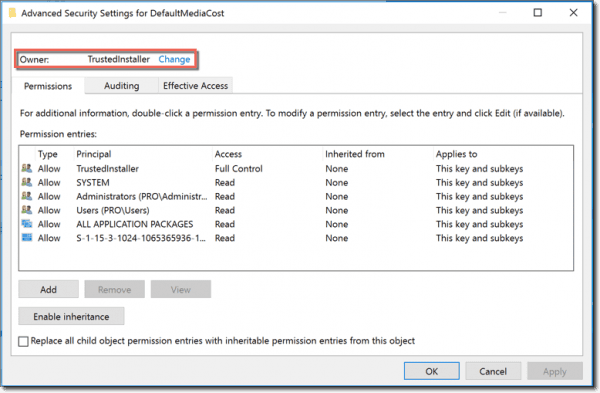
Change owner of Registry key
Type Administrators in the form field and click OK.
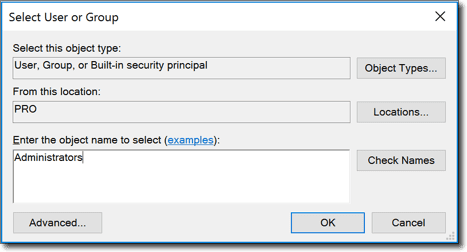
Setting Administrators as key owner
Check Replace owner on subcontainers and objects and click OK.
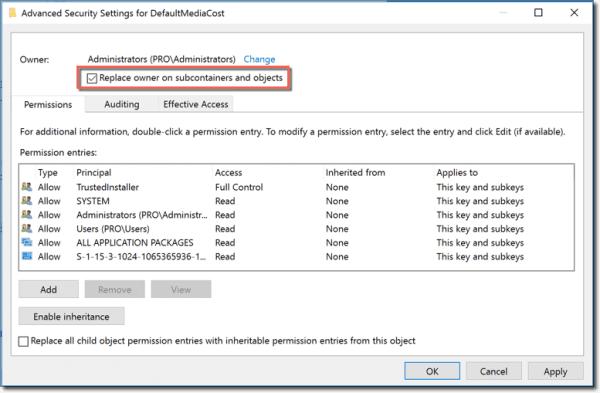
Replace owner on subcontainers and objects
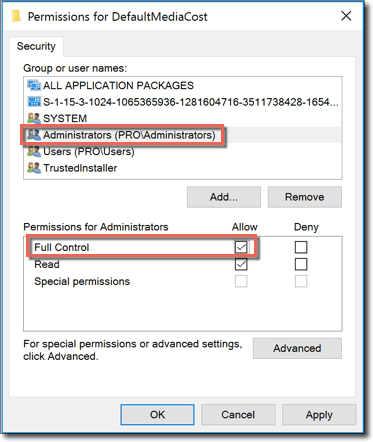
Assign Full Control permissions to Administrators
Double-click the Ethernet key and set its value to 2.
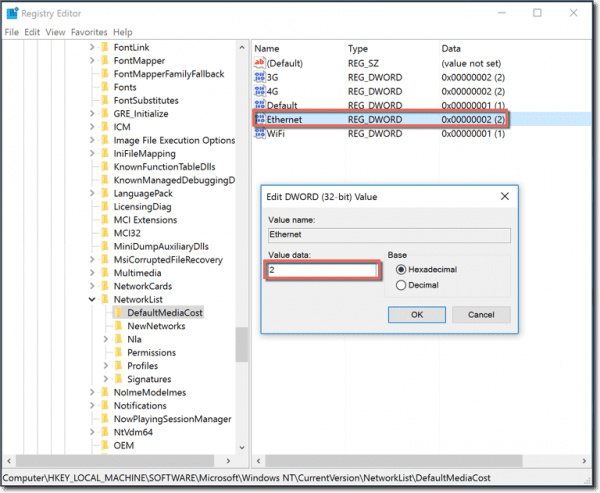
Set Ethernet connection as metered
You can set a Favorite in the Registry editor, if you want to change the key quickly later. To reset the Ethernet connection as not metered, you have to change the value to 1.
All right, this is really a lot of click-click. If you have to do this often on different machines, you can just run the PowerShell script below.
After I assign the Administrators group as the owner of the DefaultMediaCost key, I give the group full control permissions.
In the last part of the script, I check to see if the Ethernet connection is set as metered or not and then ask the user whether the current configuration should be changed.
Ethernet Connection Metered PowerShell Script
<#
.SYNOPSIS : PowerShell script to set Ethernet connection as metered or not metered
.AUTHOR : Rock Brave
.SITE : http://infosbird.com
#>
#We need a Win32 class to take ownership of the Registry key
$definition = @"
using System;
using System.Runtime.InteropServices;
namespace Win32Api
{
public class NtDll
{
[DllImport("ntdll.dll", EntryPoint="RtlAdjustPrivilege")]
public static extern int RtlAdjustPrivilege(ulong Privilege, bool Enable, bool CurrentThread, ref bool Enabled);
}
}
"@
Add-Type -TypeDefinition $definition -PassThru | Out-Null
[Win32Api.NtDll]::RtlAdjustPrivilege(9, $true, $false, [ref]$false) | Out-Null
#Setting ownership to Administrators
$key = [Microsoft.Win32.Registry]::LocalMachine.OpenSubKey("SOFTWARE\Microsoft\Windows NT\CurrentVersion\NetworkList\DefaultMediaCost",[Microsoft.Win32.RegistryKeyPermissionCheck]::ReadWriteSubTree,[System.Security.AccessControl.RegistryRights]::takeownership)
$acl = $key.GetAccessControl()
$acl.SetOwner([System.Security.Principal.NTAccount]"Administrators")
$key.SetAccessControl($acl)
#Giving Administrators full control to the key
$rule = New-Object System.Security.AccessControl.RegistryAccessRule ([System.Security.Principal.NTAccount]"Administrators","FullControl","Allow")
$acl.SetAccessRule($rule)
$key.SetAccessControl($acl)
#Setting Ethernet as metered or not metered
$path = "HKLM:\SOFTWARE\Microsoft\Windows NT\CurrentVersion\NetworkList\DefaultMediaCost"
$name = "Ethernet"
$metered = Get-ItemProperty -Path $path | Select-Object -ExpandProperty $name
Clear
if ($metered -eq 1) {
$SetMetered = Read-Host "Ethernet is currently not metered. Set to metered? (y/n)"
if ($SetMetered -eq "y") {
New-ItemProperty -Path $path -Name $name -Value "2" -PropertyType DWORD -Force | Out-Null
Write-Host "Ethernet is now set to metered."
} else {
Write-Host "Nothing was changed."
}
} elseif ($metered -eq 2) {
$SetMetered = Read-Host "Ethernet is currently metered. Set to not metered? (y/n)"
if ($SetMetered -eq "y") {
New-ItemProperty -Path $path -Name $name -Value "1" -PropertyType DWORD -Force | Out-Null
Write-Host "Ethernet is now set as not metered."
} else {
Write-Host "Nothing was changed."
}
}